Once upon a time I was feeling adventurous and offered a fellow developer to migrate his website from a bunch of hand-written HTML pages to Jekyll.
He’s a Windows user, so I had to make sure that writing, previewing and building will work there with minimal effort.
I encountered a couple of problems during the process, so I decided to document it step by step for future reference.
Install Ruby + Devkit
RubyInstaller is a project that makes working with Ruby on Windows less painful.
For this post I used Ruby+Devkit 2.4.4-2 (x64) — the recommended version which was highlighted in bold on the page.
The GUI part of the installation is pretty straightforward, all parameters set to default values. After that, a command-line installer appears.
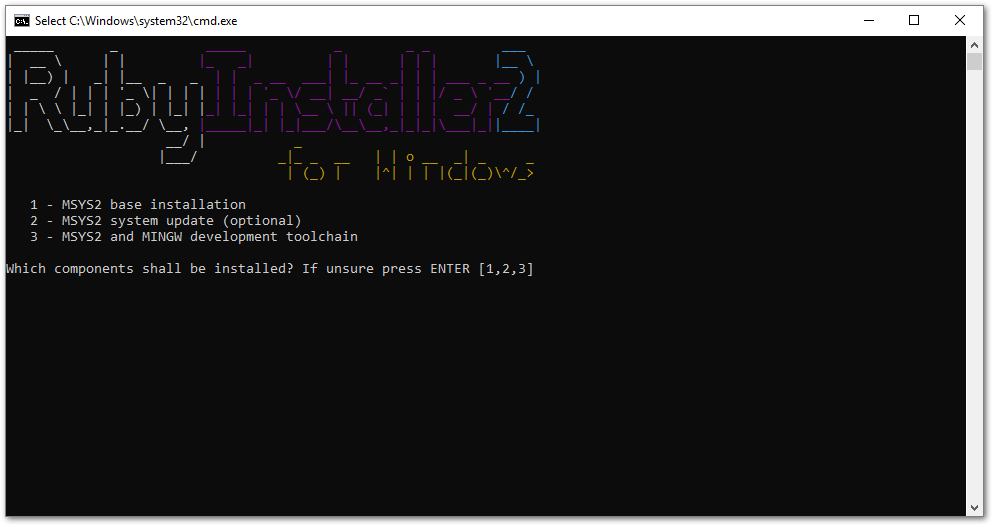
Here I had some trouble: it looked like 1,2,3
is a preselected option, but simply pressing ENTER
didn’t do anything. I had to manually type 1,2,3
and then press ENTER
.
If you wonder what these three options mean, I found this answer pretty clear.
The process takes some time and finishes with a similar screen, but the preselected option is now empty because all three components are already installed:
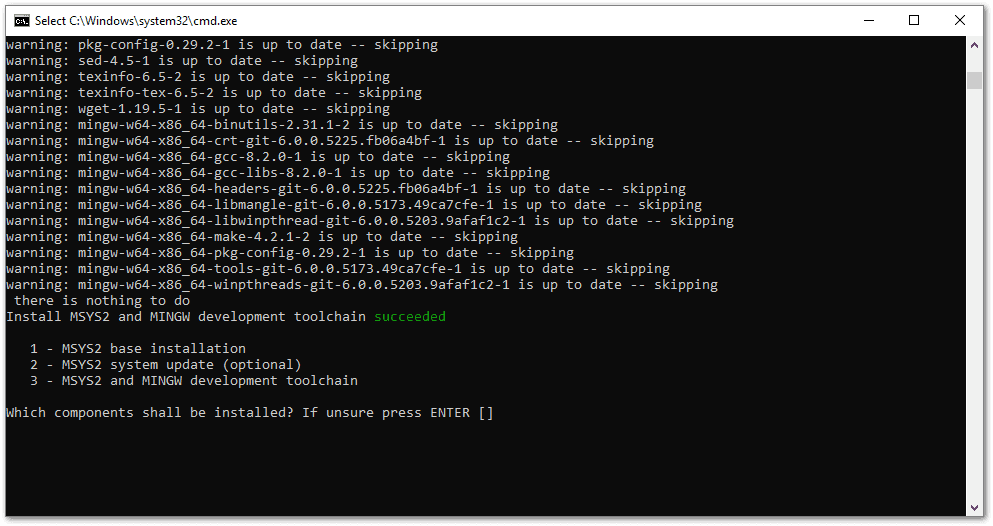
One more trouble here — I had to press ENTER
two or three times for the window to go away.
Install Jekyll
For the remaining steps I used Git bash, but Windows command prompt is also fine.
First, let’s check that Ruby is working.
$ ruby -v
ruby 2.4.4p296 (2018-03-28 revision 63013) [x64-mingw32]
Install gems as written in official Jekyll on Windows guide. For some reason I had no console output when the operation was in progress, it all appeared at the end — so don’t worry if you don’t see anything for a couple of minutes.
Note that some lines from the output are omitted and replaced with ...
$ gem install jekyll bundler
Successfully installed public_suffix-3.0.3
Successfully installed addressable-2.5.2
...
Done installing documentation for bundler after 11 seconds
26 gems installed
No errors so far. Let’s create a new Jekyll project called foo
:
$ jekyll new foo
Running bundle install in C:/Users/User/foo...
Bundler: Fetching gem metadata from https://rubygems.org/............
Bundler: Fetching gem metadata from https://rubygems.org/..
Bundler: Resolving dependencies...
...
Bundler: Bundle complete! 5 Gemfile dependencies, 33 gems now installed.
Bundler: Use `bundle info [gemname]` to see where a bundled gem is installed.
New jekyll site installed in C:/Users/User/foo.
Also no errors. However, there is one more important step…
Fix LiveReload
I’ve read about some LiveReload problems on Windows before, but this one caught me off guard:
$ cd foo
$ jekyll serve --livereload
Configuration file: C:/Users/User/foo/_config.yml
Source: C:/Users/User/foo
Destination: C:/Users/User/foo/_site
Incremental build: disabled. Enable with --incremental
Generating...
Jekyll Feed: Generating feed for posts
done in 0.49 seconds.
Auto-regeneration: enabled for 'C:/Users/User/foo'
Unable to load the EventMachine C extension; To use the pure-ruby reactor, require 'em/pure_ruby'
C:/Ruby24-x64/lib/ruby/gems/2.4.0/gems/eventmachine-1.2.7-x64-mingw32/lib/rubyeventmachine.rb:2:in `require': cannot load such file -- 2.4/rubyeventmachine (LoadError)
...
A quick Google search revealed that eventmachine
gem can be reinstalled with --platform=ruby
.
Doesn’t sound like rocket science. Let’s try it:
$ gem uninstall eventmachine
ERROR: While executing gem ... (Gem::DependencyRemovalException)
Uninstallation aborted due to dependent gem(s)
Come on, I simply want to reinstall one gem! Java dependency management spoiled me too much. But wait, they should have this parameter…
$ gem uninstall eventmachine --force
Successfully uninstalled eventmachine-1.2.7-x64-mingw32
Now better install the good version before anyone notices.
$ gem install eventmachine --platform ruby
Temporarily enhancing PATH for MSYS/MINGW...
Building native extensions. This could take a while...
Successfully installed eventmachine-1.2.7
Parsing documentation for eventmachine-1.2.7
Installing ri documentation for eventmachine-1.2.7
Done installing documentation for eventmachine after 5 seconds
1 gem installed
And the final attempt:
$ jekyll serve --livereload
Configuration file: C:/Users/User/foo/_config.yml
Source: C:/Users/User/foo
Destination: C:/Users/User/foo/_site
Incremental build: disabled. Enable with --incremental
Generating...
Jekyll Feed: Generating feed for posts
done in 0.371 seconds.
Auto-regeneration: enabled for 'C:/Users/User/foo'
LiveReload address: http://127.0.0.1:35729
Server address: http://127.0.0.1:4000/
Server running... press ctrl-c to stop.
Now the website owner can proudly update and rebuild everything on his own, enjoying Jekyll developer features even on Windows.
Update from 2020: I’ve got an email from a reader (thanks Jacob!) that LiveReload doesn’t work if there is no <head>
tag in the document, so this also may be helpful for someone. Relevant GitHub discussion